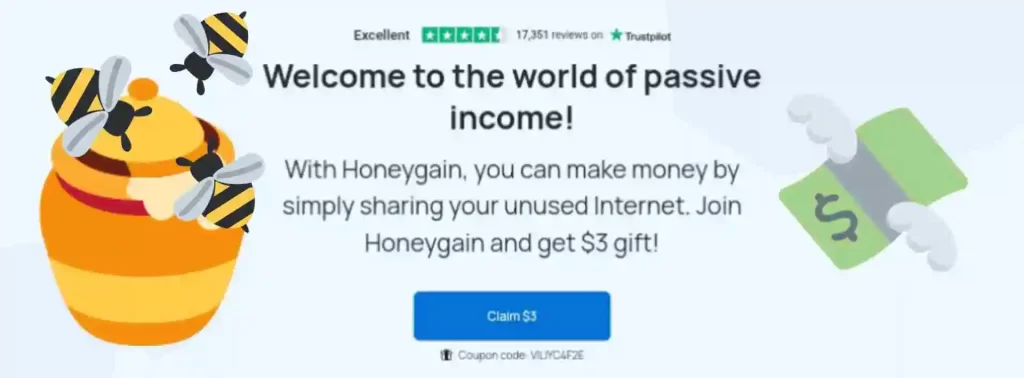
MySQL is one of the most popular open-source database management systems. PHP is a widely-used programming language for web development. In this article, we will show how to connect to a MySQL database with PHP. We will use PHP and MySQLi, which is a MySQL extension for PHP.
MySQLi is a MySQL extension for PHP that provides an interface for interacting with MySQL databases. It offers several benefits, including:
Before connecting to your MySQL database, ensure that MySQLi is installed and enabled on your server. To verify this:
phpinfo()
function.If MySQLi is not enabled, follow these steps:
php.ini
file.extension=mysqli
line.Create a new MySQL database using phpMyAdmin or the MySQL command line tool:
Alternatively, use the MySQL command line tool:
Bash
mysql -u root -p
CREATE DATABASE mydatabase;
CREATE USER 'myuser'@'%' IDENTIFIED BY 'mypassword';
GRANT ALL PRIVILEGES ON mydatabase.* TO 'myuser'@'%';
FLUSH PRIVILEGES;
Create a new PHP file (db.php
) to store your database connection settings:
PHP
// db.php
$host = 'localhost';
$username = 'myuser';
$password = 'mypassword';
$database = 'mydatabase';
Replace the placeholders with your actual database credentials.
Use the mysqli
constructor to establish a connection:
PHP
// db.php (continued)
$conn = new mysqli($host, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
This code creates a new MySQLi connection and checks for any errors.
Use the $conn
object to perform database queries:
PHP
// db.php (continued)
$query = "SELECT * FROM mytable";
$result = $conn->query($query);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo $row["column_name"] . "<br>";
}
}
This example executes a SELECT query and fetches the results.
Close the MySQLi connection when finished:
PHP
// db.php (continued)
$conn->close();
Connecting to a MySQL database with PHP and MySQLi is a straightforward process. By following these steps and adhering to best practices, you can ensure a secure and efficient database connection for your web application.
By mastering MySQLi connections, you’ll be able to create robust and data-driven web applications with PHP and MySQL.