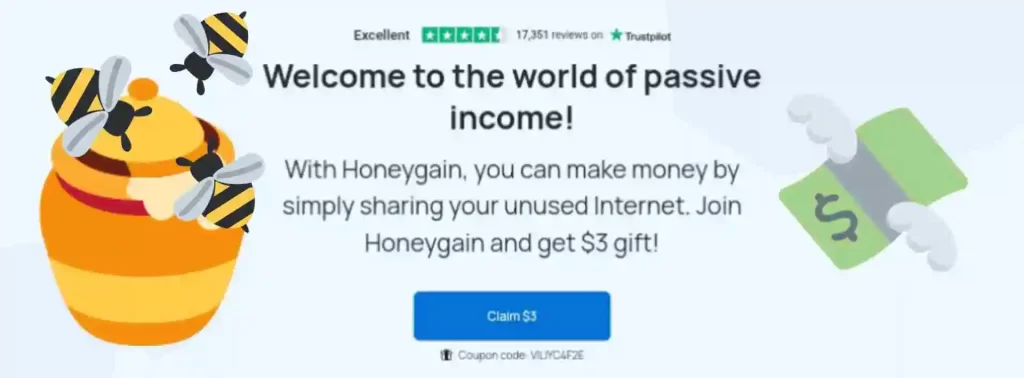
A Shell Script is a sets of Unix/Linux Commands written in a text file and with optional .sh extension to perform programmatic tasks in Unix/Linux Operating System. The Shell Scripts can be run in Command Line Interface and used for Automation.
This is the first line of a shell script that tells the operating system which interpreter to use to execute the script. For example, #!/bin/bash indicates that the script should be executed using the Bash shell.
#!/bin/bash
echo "This is example of shebang"
Comments in a shell script are lines that are ignored by the interpreter and are used to provide information or explain the code. Comments start with a # character.
Variables in shell scripts are used to store data that can be used later in the script. Variables can be assigned values using the = operator. For example, NAME=”John”.
#!/bin/bash
NAME="Viliyam"
echo "Hello $NAME"
The input can be taken by using read Command or from the Commad-Line Arguments or from the files.
The output can be displayed on the console or can be saved in a file.
#!/bin/bash
# Take input from user
echo "Enter your name"
read NAME
echo "Enter your age"
read AGE
# Display Output to console
echo "Name :$NAME, Age :$AGE"
# Save Output to file
echo "Name :$NAME, Age :$AGE" >> data.txt
Control flow statements in shell scripts are used to control the order in which commands are executed.
There are several control flow statements that you can use to control the flow of execution in your scripts. These include:
These are used to conditionally execute code based on a specific condition. The basic syntax is:
if condition
then
commands
else
commands
fi
These are used to iterate over a set of values and perform a set of commands for each value. The basic syntax is:
for variable in values
do
commands
done
These are used to repeatedly execute a set of commands while a specific condition is true. The basic syntax is:
while condition
do
commands
done
These are similar to while loops, but they execute the commands until a specific condition is true. The basic syntax is:
until condition
do
commands
done
These are used to conditionally execute code based on the value of a variable. The basic syntax is:
case variable in
value1)
commands;;
value2)
commands;;
*)
commands;;
esac
Function is a block of code that performs a specific task. Functions help to organize code and reduce redundancy by allowing you to reuse the same block of code in multiple places within a script.
function functionName() {
# bunch of codes
}
# call a function
functionName
Let’s create one function to understand more.
function greet() {
echo "Hello, $1 let's learn shell script with easymux!"
}
greet "John"
greet "Viliyam"
greet "Pritesh"
Shell scripts can include error handling code to handle errors that may occur during execution.
There are several techniques that we can use to handle errors in shell script.
Most shell commands return a numeric return code. 0 return code indicates that command is successfully executed. If return code is non-zero means command is failed.
Adding retuen code statments on successfull execution or failer of shell script is best practice in writing shell script.
set -e
option:This option tells the shell to exit immediately if any command in the script fails. This can be useful to catch errors early and prevent the script from continuing to run when there is a problem. You can set this option at the beginning of your script like this:
trap
command:The trap
command allows you to specify a command or script to run when a signal is received. This can be used to catch errors and perform cleanup operations. For example, you can use the trap
command to run a cleanup script when the script is terminated:
set -o pipefail
option:This option sets the exit code of a pipeline to the status of the last (rightmost) command to exit with a non-zero status, or to zero if all commands of the pipeline exit successfully.
In Linux and macOS, there are three types of permissions: read, write, and execute. In order to run or execute a shell script the execute permission must be given to the file.
Before executing a shell script, the script must have execution permissions. This can be set using the chmod command.
chmod 777 shellscript.sh
# or
chmod +x shellscript.sh
# To run...
./shellscript.sh
Here is an example of a simple shell script that takes a user’s name as input and outputs a greeting:
#!/bin/bash
# Take input from user
echo "Enter your name"
read NAME
echo "Enter your age"
read AGE
# Check name is not empty
if [ -z $NAME ];
then
echo "Name should not be empty"
# Exit from script if name is empty.
exit 1
fi
# Check age is number or not
if [ "$AGE" -eq "$AGE" 2>/dev/null ];
then
# Display Output to console
echo "$NAME is $AGE years old"
# Save Output to file
echo "Name :$NAME, Age :$AGE" >> data.txt
# Exit with return code 0 of success
exit 0
else
echo "Age must be number of years of your age."
# Exit from script if age is not a number.
exit 1
fi
Overall, shell scripts are a powerful tool for automating tasks in a Unix/Linux command-line enviroment and are widely used in system administration, software development, and data processing.